Overview
M0 is the universal stablecoin platform. With M0, developers can build their own application-specific digital dollars and embed those into any use case.
Stablecoins as a concept emerged a decade ago with the simple goal of representing a dollar on the blockchain. The initial wave of products in the sector helped settle trillions of dollars in crypto capital markets transactions over the last number of years.
But money is critical infrastructure, not a product. The initial generation of stablecoins was too simplistic in its design, and a new financial system simply can't be rewritten on top of it. M0 was founded to reconstruct the monetary stack from first principles, enabling a new wave of digital dollar applications to be built.
The M0 Model
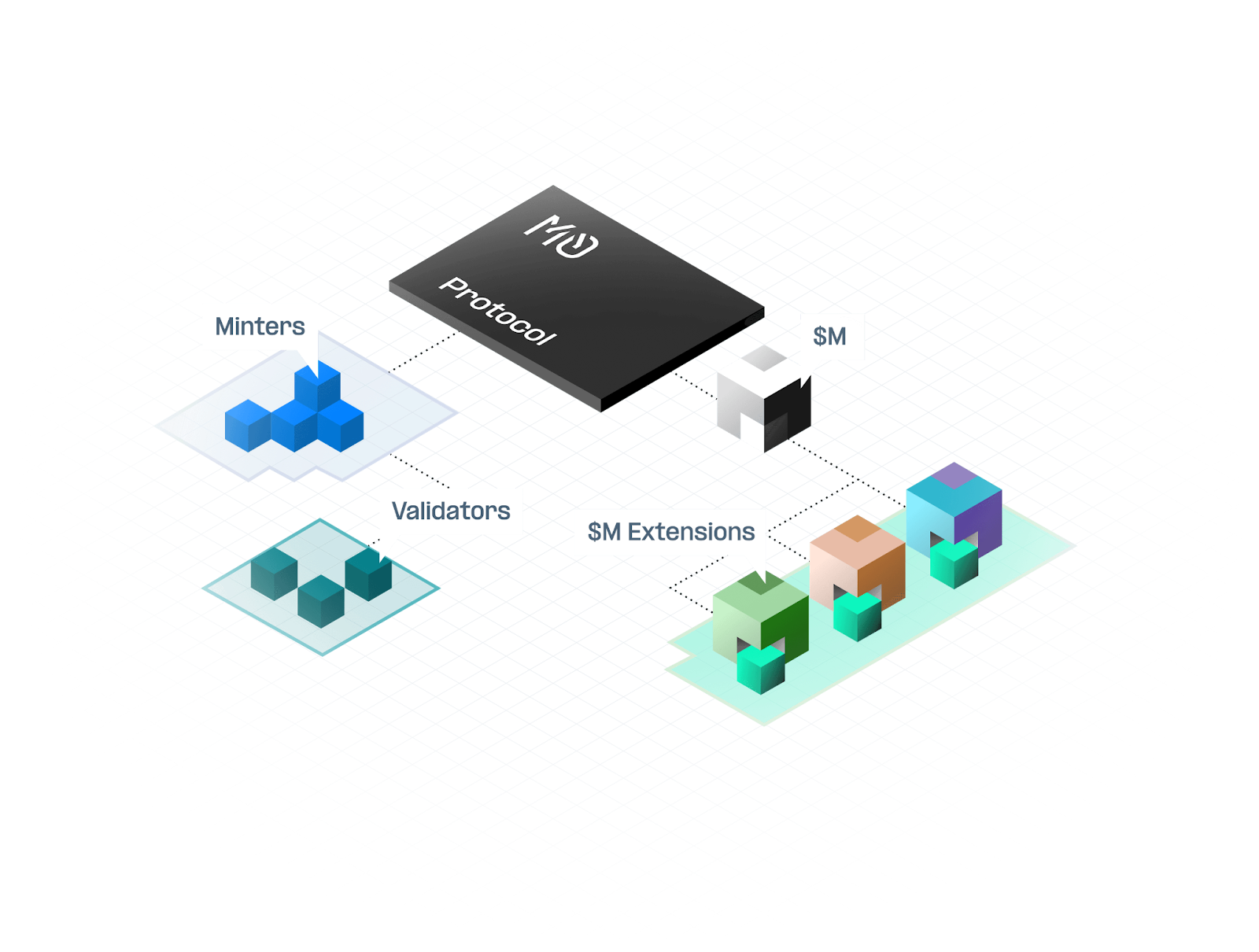
Application developers value control and the ability to customize their technology stack for their context. One can think of fintech as the customization of dollars into a particular form-factor that favors the use case. Stablecoins allow for onchain programmability of digital dollar behavior. But one-size-fits-all stablecoins issued by centralized actors do not really allow for programmability. The M0 platform was built to help developers create and program their own bespoke digital dollars.
How to Use This Documentation Portal
Below is an overview of how this documentation portal is organized: